Adding a new OAuth provider
Let's walk through adding Facebook as a new OAuth provider
- Define the new provider string in the
app/services/auth/types.ts
export const GOOGLE_AUTH_PROVIDER = "google";
export const GITHUB_AUTH_PROVIDER = "github";
+export const FACEBOOK_AUTH_PROVIDER = "facebook";
export type ProviderName =
| typeof GOOGLE_AUTH_PROVIDER
| typeof GITHUB_AUTH_PROVIDER
+| typeof FACEBOOK_AUTH_PROVIDER;
- Register your Facebook app to obtain the
OAUTH_APP_ID
andOAUTH_APP_SECRET
and add them to your.env
+ FACEBOOK_OAUTH_APP_ID=1234567890123456
+ FACEBOOK_OAUTH_APP_SECRET=abcdef0123456789abcdef0123456789
Optionally, add the environment variables to the env.server.ts
parsing so they are type-safe at runtime
- Create a new file in the
app/services/auth/providers
calledfacebook.server.ts
export const facebookAuthProvider: AuthProvider = {
strategy: new OAuth2Strategy(
{
authorizationURL: "https://www.facebook.com/v11.0/dialog/oauth",
tokenURL: "https://graph.facebook.com/v11.0/oauth/access_token",
clientID: process.env.FACEBOOK_OAUTH_APP_ID,
clientSecret: process.env.FACEBOOK_OAUTH_APP_SECRET,
callbackURL: "/auth/facebook/callback",
},
// Not fully implemented for brevity
- Add your new
facebookAuthProvider
to theapp/services/auth/providers/providers.ts
export const ProviderNameSchema = z.enum([
GOOGLE_AUTH_PROVIDER,
GITHUB_AUTH_PROVIDER,
+ FACEBOOK_AUTH_PROVIDER,
]);
export const providers: Record<ProviderName, AuthProvider> = {
[GOOGLE_AUTH_PROVIDER]: googleAuthProvider,
[GITHUB_AUTH_PROVIDER]: githubAuthProvider,
+ [FACEBOOK_AUTH_PROVIDER]: facebookAuthProvider,
};
- Add a new button to
app/components/auth/social-auth-buttons.tsx
and voila!
const GithubAuthButton = () => {
return (
<SocialAuthButton provider={"github"} title={"GitHub"} icon={GithubIcon} />
);
};
+const FacebookAuthButton = () => {
+ return (
+ <SocialAuthButton provider={"facebook"} title={"Facebook"} icon={FacebookIcon} />
+ );
+};
export const SocialAuthButtons = ({
authProviders,
}: {
authProviders: ProviderName[];
}) => {
return (
<div className={`grid gap-6`}>
{authProviders.includes("google") && <GoogleAuthButton />}
{authProviders.includes("github") && <GithubAuthButton />}
+ {authProviders.includes("facebook") && <FacebookAuthButton />}
</div>
);
};
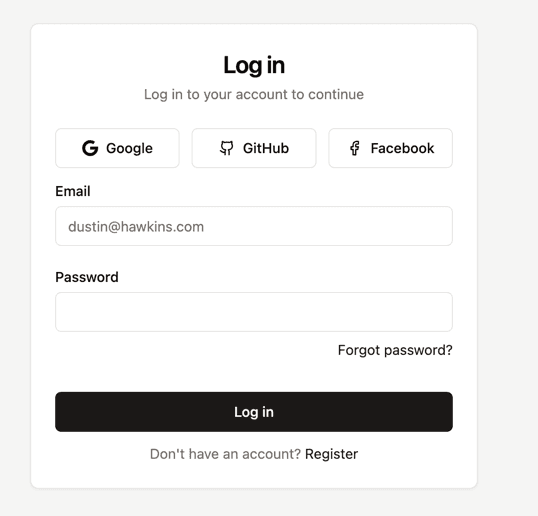